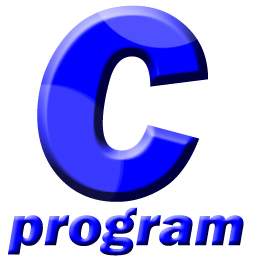
#include < stdio.h>
void trace(int arr[][10], int m, int n);
void main()
{
int array1[10][10], array2[10][10], arraysum[10][10],
arraydiff[10][10];
int i, j, m, n, option;
printf("Enter the order of the matrix array1 and array2 \n" );
scanf("%d %d" , &m, &n);
printf("Enter the elements of matrix array1 \n" );
for (i = 0; i < m; i++)
{
for (j = 0; j < n; j++)
{
scanf("%d" , &array1[i][j]);
}
}
printf("MATRIX array1 is \n" );
for (i = 0; i < m; i++)
{
for (j = 0; j < n; j++)
{
printf("%3d" , array1[i][j]);
}
printf("\n" );
}
printf("Enter the elements of matrix array2 \n" );
for (i = 0; i < m; i++)
{
for (j = 0; j < n; j++)
{
scanf("%d" , &array2[i][j]);
}
}
printf("MATRIX array2 is \n" );
for (i = 0; i < m; i++)
{
for (j = 0; j < n; j++)
{
printf("%3d" , array2[i][j]);
}
printf("\n" );
}
printf("Enter your option: 1 for Addition and 2 for Subtraction \n" );
scanf("%d" , &option);
switch (option)
{
case 1:
for (i = 0; i < m; i++)
{
for (j = 0; j < n; j++)
{
arraysum[i][j] = array1[i][j] + array2[i][j];
}
}
printf("Sum matrix is \n" );
for (i = 0; i < m; i++)
{
for (j = 0; j < n; j++)
{
printf("%3d" , arraysum[i][j]) ;
}
printf("\n" );
}
trace (arraysum, m, n);
break;
case 2:
for (i = 0; i < m; i++)
{
for (j = 0; j < n; j++)
{
arraydiff[i][j] = array1[i][j] - array2[i][j];
}
}
printf("Difference matrix is \n" );
for (i = 0; i < m; i++)
{
for (j = 0; j < n; j++)
{
printf("%3d" , arraydiff[i][j]) ;
}
printf("\n" );
}
trace (arraydiff, m, n);
break;
}
}
/* Function to find the trace of a given matrix and print it */
void trace (int arr[][10], int m, int n)
{
int i, j, trace = 0;
for (i = 0; i < m; i++)
{
for (j = 0; j < n; j++)
{
if (i == j)
{
trace = trace + arr[i][j];
}
}
}
printf("Trace of the resultant matrix is = %d\n" , trace);
}
Output
Enter the order of the matrix array1 and array2
3 3
Enter the elements of matrix array1
2 3 4
7 8 9
5 6 8
MATRIX array1 is
2 3 4
7 8 9
5 6 8
Enter the elements of matrix array2
3 3 3
3 4 6
8 4 7
MATRIX array2 is
3 3 3
3 4 6
8 4 7
Enter your option: 1 for Addition and 2 for Subtraction
1
Sum matrix is
5 6 7
10 12 15
13 10 15
Trace of the resultant matrix is = 32
Enter the order of the matrix array1 and array2
3 3
Enter the elements of matrix array1
10 20 30
15 18 20
12 14 16
MATRIX array1 is
10 20 30
15 18 20
12 14 16
Enter the elements of matrix array2
1 5 9
10 15 14
9 12 13
MATRIX array2 is
1 5 9
10 15 14
9 12 13
Enter your option: 1 for Addition and 2 for Subtraction
2
Difference matrix is
9 15 21
5 3 6
3 2 3
Trace of the resultant matrix is = 15
For More Details Please Visit Ictjobs.info
No comments:
Post a Comment