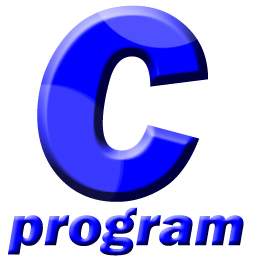
#include< stdio.h> #include< string.h> #include< sys/types.h> #include< sys/socket.h> #include< sys/ioctl.h> #include< netinet/in.h> #include< net/if.h> #include< unistd.h> #include< arpa/inet.h> int main() { int n; struct ifreq ifr; char array[] = "eth0"; n = socket(AF_INET, SOCK_DGRAM, 0); //Type of address to retrieve - IPv4 IP address ifr.ifr_addr.sa_family = AF_INET; //Copy the interface name in the ifreq structure strncpy(ifr.ifr_name , array , IFNAMSIZ - 1); ioctl(n, SIOCGIFADDR, &ifr); close(n); //display result printf("IP Address is %s - %s\n" , array , inet_ntoa(( (struct sockaddr_in *)&ifr.ifr_addr )->sin_addr) ); return 0; }
Output
IP Address is eth0 - 192.168.225.135
For More Details Please Visit Ictjobs.info
No comments:
Post a Comment